Story
Icebox is a demo project that helped me with learning more about C++. At first, this project used the Flax Engine, but after some time I decided to switch to Unreal Engine 5.
Right now the player's objective is to accept a mission and store the boxes in the space station receiver as quickly as possible after doing that they receive a score.
Features:
For the Future
I'd like to add:
- Sandbox Mode
- Multiplayer CO-OP
- Economy
- Tractor SpaceShip to move heavier containers
- Upgrades for the player and the tractor spaceship
- A new space station
Features
Dynamic Box Spawner
can spawn small boxes inside a bounding box or spawn Containers to be attached to the Cargo Spaceship. Containers are used to transport boxes, each container has a bounding box and a BoxSpawnerComponent.
To spawn the boxes inside the container, we can divide the bounding box width/height/depth with the box size to get how many boxes can be spawned. To spawn boxes with different sizes, bigger boxes are spawned first, after that when a small box wants to spawn it will check for a collision with a bigger box. If a collision is not detected then it's safe to spawn.
A small box has a size of 30 units, a container has a size of 90 so it can spawn up to 27 small boxes.
In Figure 2 you can see the BoxSpawnerComponent has 2 attributes: SpaceBetweenBoxes(int), and ObjectsToSpawn(TMap). This example will spawn 1 Fuel Box, 9 Timer Boxes and 10 Stability Boxes, Figure 1 can prove that.
DISCLAIMER: Container mesh visibility is off to show the boxes inside the container!
![]() |
![]() |
As you can see in Figure 3 the containers are attached to the Cargo Spaceship, in this example it spawned 10 Fuel Boxes, 95 Stability Boxes and 95 Timer Boxes.
The Cargo Spaceship has 10 attach points so it can carry up to 270 small boxes in total.
![]() |
![]() |
Gravity Gun
first prototype was in Flax Engine but after some time I switched to Unreal Engine 5.
The gravity gun has the ability to move/rotate objects and give them an impulse.
To help the player the GravGun has a HUD that can display:
- Box Speed: White = Safe to move | Yellow = Dangerous Speed | Red = Critical Speed, taking damage
- Box Timer: Green = Timer > 60s | Orange = Timer < 60s | Red = Timer < 10s
- Box Distance
Final Version:
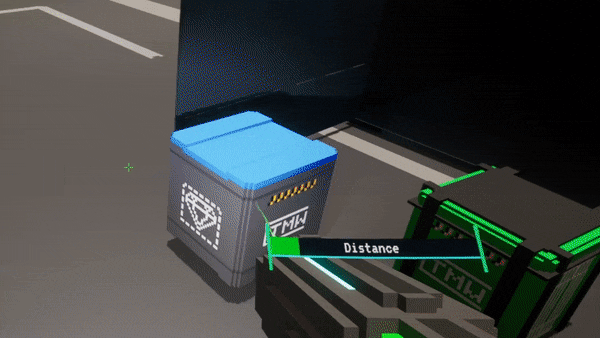
Cargo Boxes Behaviours
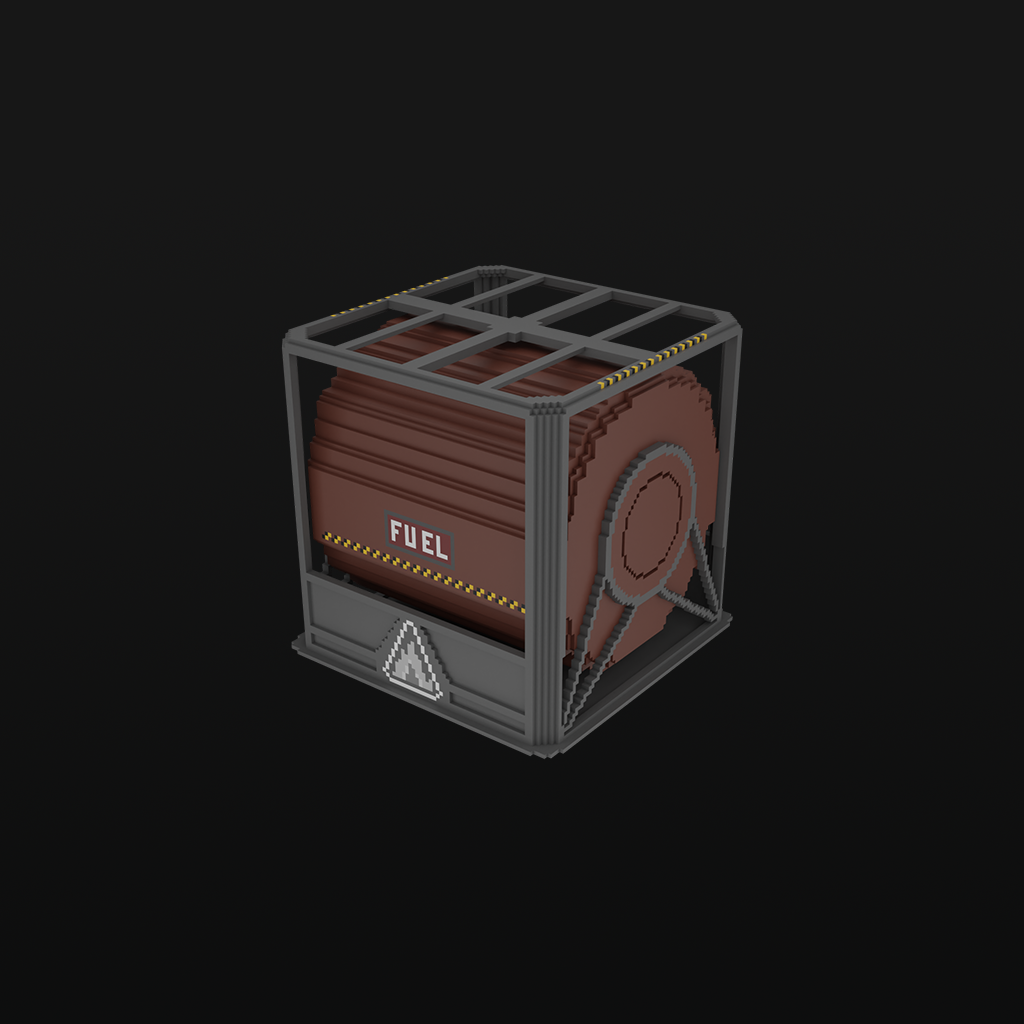
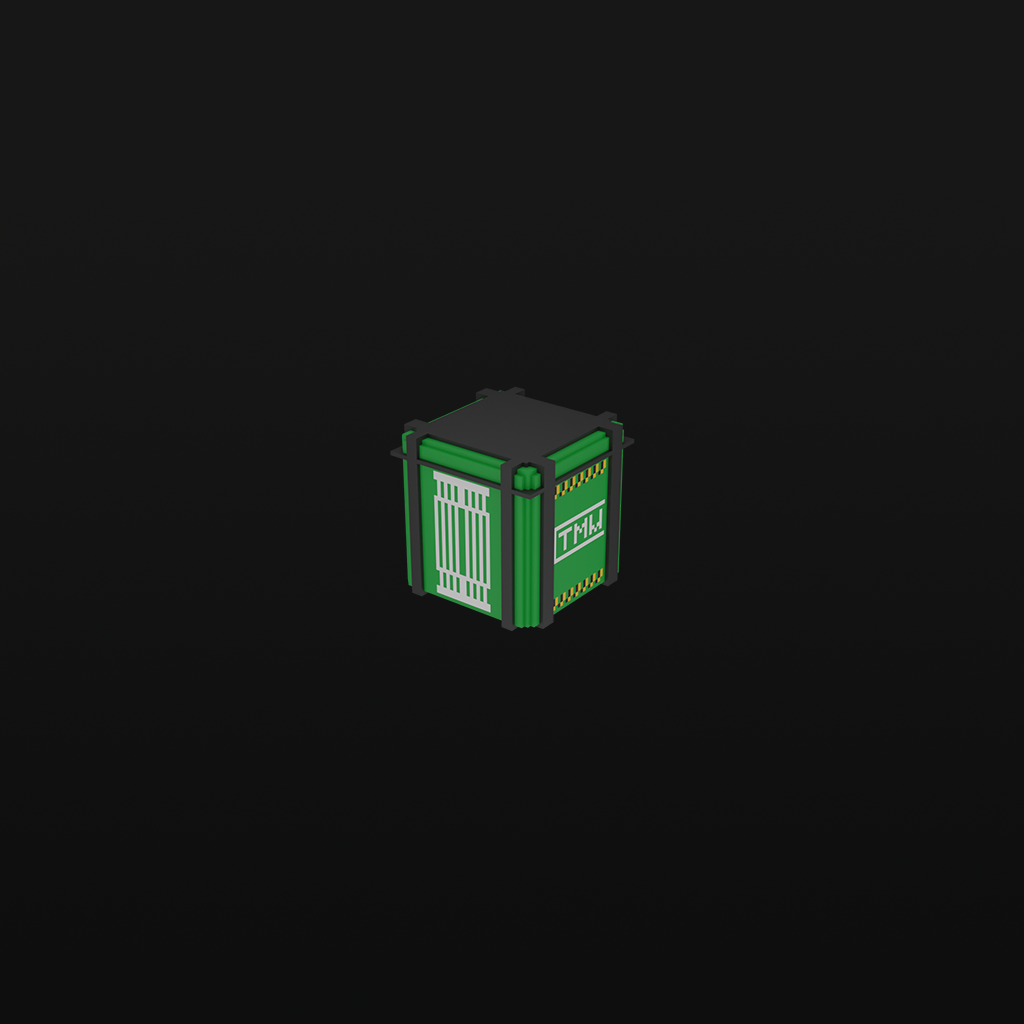
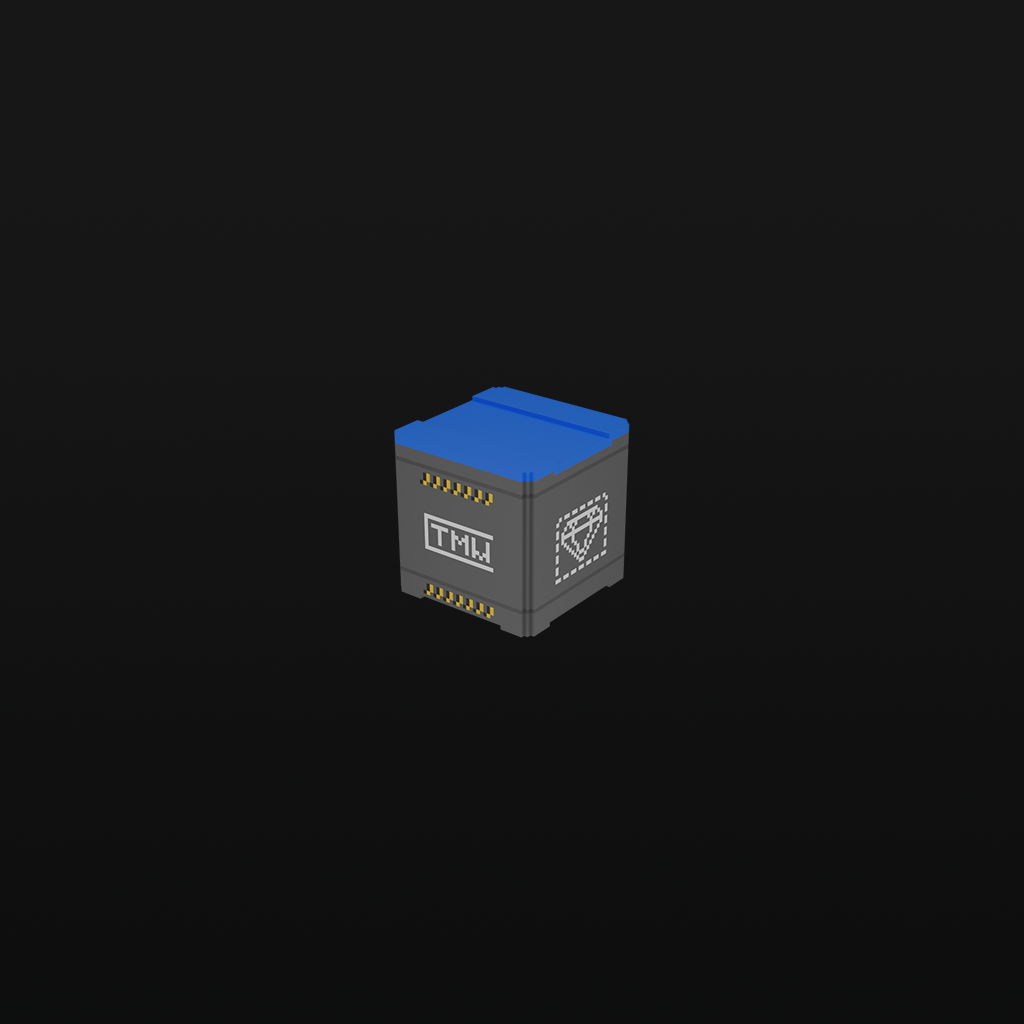
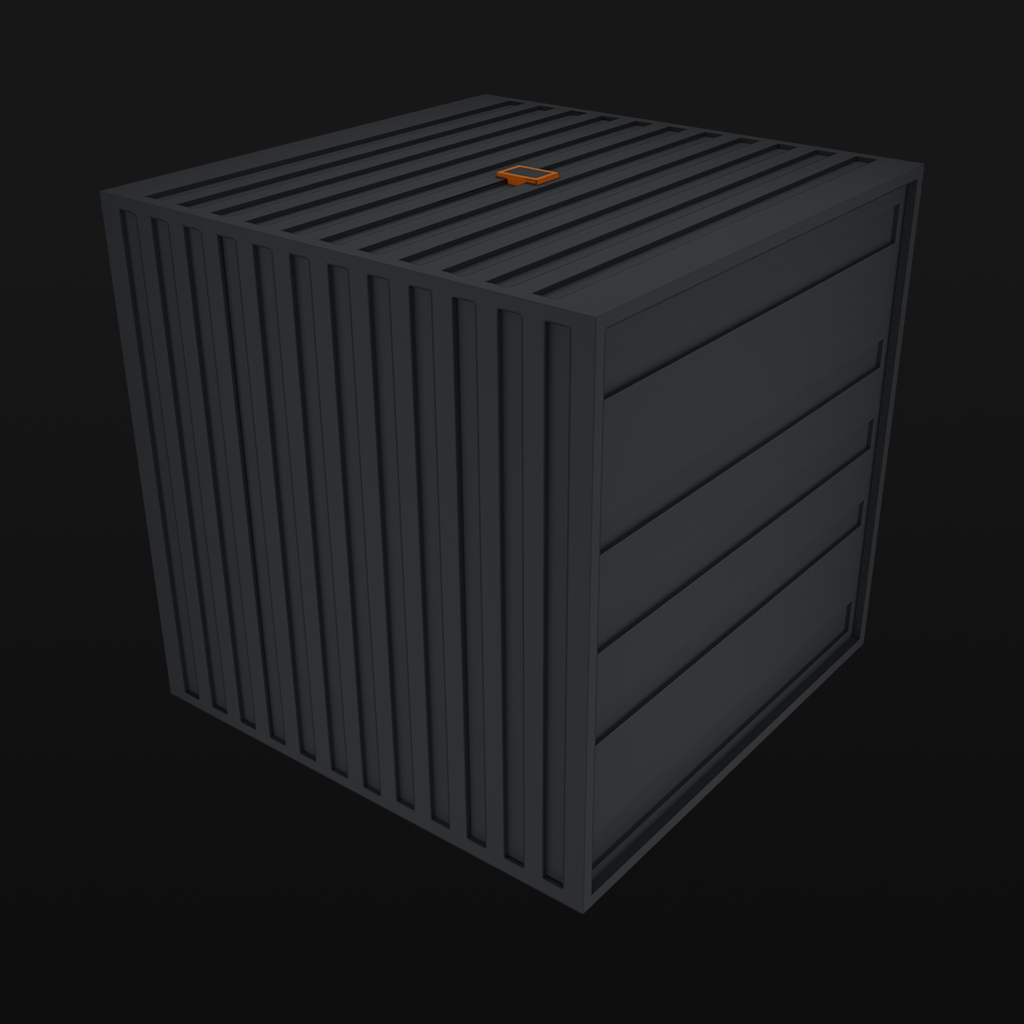
Mini Mission System
When the game mode starts depending on the parameters it will generate X missions of Y Difficulty. In this case, it will create 3 missions for regular, medium, and hard difficulty.
To achieve this I created a mission row template that can be added to the missions scroll box with the mission information.
The player can accept the mission, finish the mission and filter the mission by difficulty or by completion.
The mission content row ui has 2 parameters: MissionData - has all the information about the mission; MissionScrollBox - Used by the accept/finish button to enable / disable other mission rows.
It can display: mission id, mission from location, mission difficulty, points, boxes to move, and if the mission is finished.
AI Follow Vector Path
To move the cargo spaceship I created a simple "AI" that contains an array of a custom struct FSpaceShipPath
USTRUCT(BlueprintType) struct FSpaceShipPath { GENERATED_BODY() UPROPERTY(EditAnywhere, BlueprintReadOnly) FVector Position; // The position to move the space ship. UPROPERTY(EditAnywhere, BlueprintReadOnly) bool ToMove = true; // Should the spaceship keep moving? UPROPERTY(EditAnywhere, BlueprintReadOnly) bool IsLoadUnloadArea = false; // If it's a load / unload area it will set the ship lights to green, so the player knows it's safe to load / unload. };
Player Movement
The player movement uses the default player character from UE with some modifications made by me, for example: 0G Movement, Move from Gravity Active to 0G, Grabbed Object Mass can change the max player speed
In 0G the player can move forward / backward, strafe up/down/right/left, roll right/left, and finally look around.